Decoding Python Magic -> __neg__ and __pos__
Let’s see how unary — and + works.
Welcome to another installment of my ongoing ‘Decoding Python Magic’ series. Today, I’m excited to unveil the inner workings of unary operators such as -
and +
in Python.
__neg__
Indeed, the usage of these operators is straightforward and intuitive, mirroring their real-world counterparts. Let’s delve into a practical example by creating a Vector
class to demonstrate their usage.
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __neg__(self):
return Vector(-self.x, -self.y)
def __add__(self, other):
return Vector(self.x + other.x, self.y + other.y)
def __repr__(self):
return f"Vector({self.x}, {self.y})"
v = Vector(1, 2)
v + (-v)
Vector(0, 0)
__pos__
In our Vector
class, we'll redefine the behavior of the unary plus operator (+
) to calculate the positive magnitude of the vector, showcasing the versatility of Python's magic methods.
import math
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __neg__(self):
return Vector(-self.x, -self.y)
def __add__(self, other):
return Vector(self.x + other.x, self.y + other.y)
def __pos__(self):
magnitude = math.sqrt(self.x**2 + self.y**2)
return Vector(magnitude, 0)
def __repr__(self):
return f"Vector({self.x}, {self.y})"
v = Vector(1, 2)
print(+v)
Vector(2.23606797749979, 0)
Conclusion
the __neg__
and __pos__
methods in Python provide powerful mechanisms for defining custom behavior for unary operators. By implementing these methods in your classes, you can control how objects respond to the unary negation (-
) and unary plus (+
) operators, respectively. This flexibility allows you to create more intuitive and expressive classes, making your code easier to read and maintain. Whether you're working with numbers, vectors, or other custom types.
Hope you learned something new. You can follow Rahul Beniwal for upcoming articles. Keep learning.
Other similar writings by me.
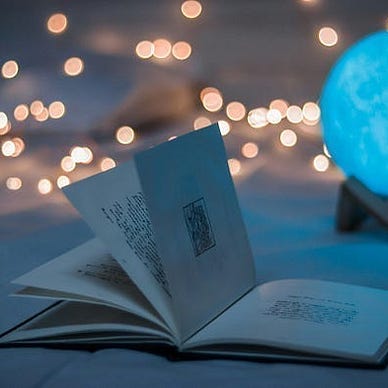







